課後作業-JavaScript 系列六:第7課 ── 資料序列化
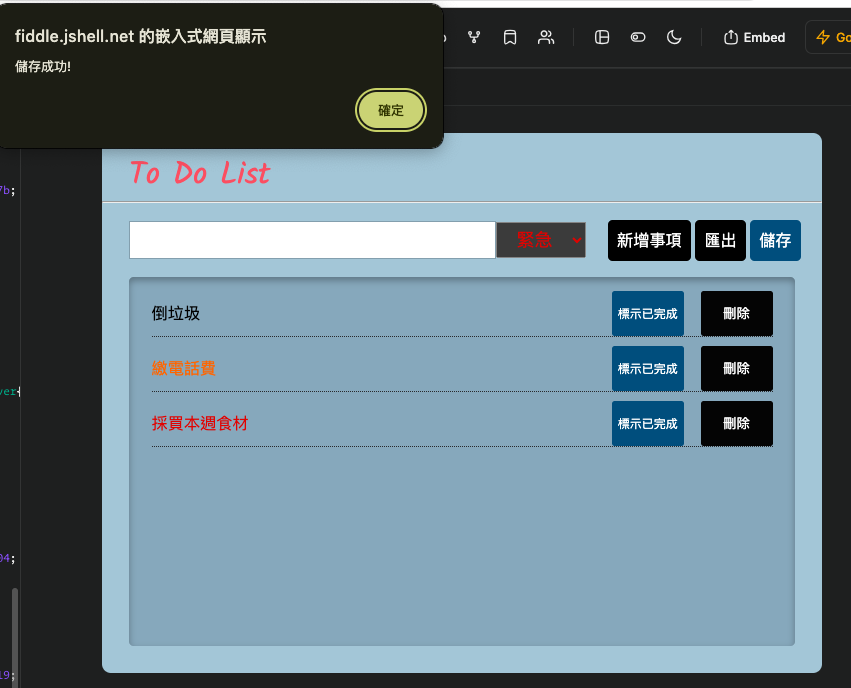
07_js_localstorage.html
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47
| <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>JavaScript 系列六:第6課 ── 認識 data model 的優點</title> <link rel="stylesheet" href="./07_js_localstorage.css"> </head> <body> <input type="text"> <button id="add_btn" onclick="add()">新增事項</button> <button id="export_btn" onclick="export_todos()">匯出</button> <button id="save_btn" onclick="save_todos()">儲存</button> <div id="root"> </div>
<script src="./07_js_localstorage.js"></script> </body> </html>
|
07_js_localstorage.js
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224
|
var todos = [];
function render_wrap(){ const body = document.querySelector('body'); const wrap = document.createElement('div'); wrap.className='wrap'; const h2 = document.createElement('h2'); h2.textContent="To Do List"; const hr = document.createElement('hr');
const input_todo = document.querySelector('input'); const add_btn = document.querySelector('#add_btn'); const export_btn = document.querySelector('#export_btn'); const save_btn = document.querySelector('#save_btn'); const select = document.createElement('select'); const option_normal = document.createElement('option'); option_normal.value = 'normal'; option_normal.textContent = '一般'; option_normal.selected = true; const option_important = document. createElement('option'); option_important.value = 'important'; option_important.textContent = '重要';
const option_urgent = document.createElement('option'); option_urgent.value = 'urgent'; option_urgent.textContent = '緊急';
const root = document.querySelector('#root');
select.append(option_normal); select.append(option_important); select.append(option_urgent); wrap.append(h2); wrap.append(hr); wrap.append(input_todo); wrap.append(select); wrap.append(add_btn); wrap.append(export_btn); wrap.append(save_btn); wrap.append(root);
body.append(wrap);
select.addEventListener('change', function () { const selectedValue = select.value; select.className = ''; if (selectedValue === 'important') { select.className += ' important'; } else if (selectedValue === 'urgent') { select.className += ' urgent'; } });
input_todo.addEventListener('keydown', function(event) { if (event.key === 'Enter') { add(); } }); }
function add() { const input_todo = document.querySelector('input'); const select_category = document.querySelector('select');
let todo = input_todo.value.trim(); let category = select_category.value; let isCompleted = false;
if(todo !== ''){ todos.push({ title: todo, category: category, isCompleted: isCompleted }); console.log(todos); render(); }else { input_todo.focus(); input_todo.placeholder = "新增待辦事項"; } input_todo.value = ''; }
function render(){ console.log('render....'); const root = document.querySelector('#root'); root.textContent=''; const div_container = document.createElement('div'); div_container.className = 'todos_container'; const ul = document.createElement('ul'); ul.className = 'todoList';
todos.forEach(function(item, index){ const li = document.createElement('li'); const span = document.createElement('span'); const toggleBtn = document.createElement('button'); toggleBtn.className = item.isCompleted ? 'btn_li btn_on' : 'btn_li btn_off'; toggleBtn.textContent = item.isCompleted ? '改為待完成' : '標示已完成'; const delBtn = document.createElement('button'); delBtn.className = "btn_li delBtn"; delBtn.textContent = '刪除';
toggleBtn.onclick = () => { item.isCompleted = !item.isCompleted; render(); }
delBtn.onclick = () => { todos = todos.filter((_, i) => i !== index); render(); };
span.textContent = item.title;
if (item.isCompleted) { const completedText = document.createElement('span'); completedText.textContent = ' (已完成)'; span.appendChild(completedText); }
li.className = item.category;
li.append(span); li.append(toggleBtn); li.append(delBtn); ul.append(li); });
div_container.append(ul); root.append(div_container); };
function export_todos() { let export_msg = ''; todos.forEach(function(item, index) { const number = index + 1; let formattedTitle = item.title; if (item.category === 'important') { formattedTitle = `*${item.title}*`; } else if (item.category === 'urgent') { formattedTitle = `**${item.title}**`; } const status = item.isCompleted ? '(已完成)' : ''; export_msg += `${number}. ${formattedTitle} ${status}\n`; }); alert(export_msg.trim()); }
function save_todos() { console.log('Saving todos...'); localStorage.setItem('todos', JSON.stringify(todos)); alert('儲存成功!'); }
function load_todos() { const savedTodos = localStorage.getItem('todos'); if (savedTodos) { todos = JSON.parse(savedTodos); render(); } } window.onload=()=>{ render_wrap(); load_todos(); };
|
07_js_localstorage.css
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143
| @import url('https://fonts.googleapis.com/css2?family=Kalam:wght@300;400;700&family=Playwrite+CU:wght@100..400&display=swap'); *{ padding: 0; margin: 0; }
.wrap{ margin: 30px auto; width: 800px; min-height: 600px; background-color: #a9c5d5; border-radius: 10px; } h2{ padding-top: 20px; padding-left: 30px; font-family: "Kalam", cursive; font-weight: 400; font-style: normal; font-size: 35px; color:#e8626b; }
input{ width: 400px; margin: 20px 0 20px 30px; padding-left: 5px; font-size: 20px; line-height: 2; border: 1px solid #889fad; color:#000; background-color: #fff;
} input:hover, input:focus { outline: none; background-color: #eaeaa9; } select{ width: 100px; height: 40px; font-size: 20px; line-height: 2; margin-right: 20px; text-align: center; } .important{ color: #ed7023; } .urgent{ color: #cf372c; }
#add_btn , #export_btn ,#save_btn{ margin-left: 5px; border: none; font-size: 18px; border-radius: 5px; background-color: #000; color: #fff; padding: 10px; } #add_btn:hover, #save_btn:hover{ cursor: pointer; background-color: #17507b; } #export_btn:hover{ cursor: pointer; background-color: #b91919; } ul{ list-style-type: square; min-height: 400px; margin: 0 30px; padding: 5px; border-radius: 5px; background-color: #8ca8ba; box-shadow: inset 0 4px 8px rgba(0, 0, 0, 0.3);
} li { display: flex; justify-content: space-between; align-items: center; margin: 10px 20px; border-bottom: 1px dotted #28292b; font-size: 18px; color: #000; }
span { display: block; word-wrap: break-word; width: 70%; margin-right: 10px;
}
.btn_li { flex-shrink: 0; width: 30%; margin-left: 10px; }
.btn_on{ color:#f00; background-color: inherit; } .btn_off{ color: #fff; background-color: #17507b; } .btn_on , .btn_off{ width:80px; height: 50px; border: none;
border-radius: 3px; padding: 5px; } .btn_on:hover , .btn_off:hover{ cursor: pointer; }
.delBtn{ width:80px; height: 50px; border: none; font-size: 15px; border-radius: 3px; background-color: #040404; color: #fff; padding: 10px; }
.delBtn:hover{ cursor: pointer; background-color: #b91919; }
|
作業取自: https://codelove.tw/@howtomakeaturn/post/NxN6yx
by 站長阿川